VirtualBox : Installing Oracle VM VirtualBox
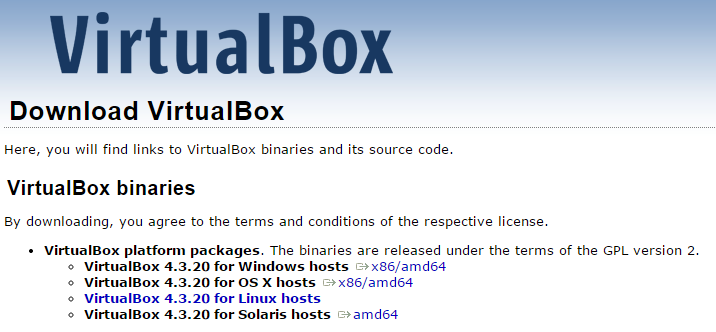
Oracle VM VirtualBox is a great virtualization software, and the best part about it is that it's free. If you have a Windows operating system and you want to explorer Linux and UNIX distributions for fun, then VirtualBox is the way to go. Here are the steps to install VirtualBox on your machine: Go to https://www.virtualbox.org/wiki/Downloads to download the latest version of VirtualBox for your operating system. 2. Double click on the .exe file that you've just downloaded 3. Click "Next" on the intro screen 4. Click "Next" on the "Custom Setup" screen 5. Click next on the second "Custom Setup" screen. 6. Click "Yes" on the "Warning: Network Interfaces" screen, don't worry your network connection will only be interrupted briefly. 7. Click "Install" in the "Ready to Install" screen 8, Click "Yes" on the "User Access Control" prompt from Windows 9. The installer wi