RadioButtonList : Bind RadioButtonList To A List Of Objects
The RadioButtonList displays a collection of radio buttons on a web page. There can only be one selection in a group of choices at a time. In this tutorial we will create a RadioButtonList and then bind it to the Categories table of the Northwind database using a DataTable.
To create a RadioButtonList control do the following:
1. Select the "RadioButtonList" control under the "Standard" control in the "Toolbox" pane on the left.
2. Drag the RadioButtonList control to a design surface
3. In the source code of the .aspx page make sure "AutoPostBack" is set to true, the source code should look like this
4. Create a connection string in the Web.Config file
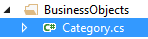
6. In the "Category.cs" file type in the following code
7. In the code behind page(.cs) page of the .aspx page, type in the following lines at the top to use the following libraries
To create a RadioButtonList control do the following:
1. Select the "RadioButtonList" control under the "Standard" control in the "Toolbox" pane on the left.
2. Drag the RadioButtonList control to a design surface
3. In the source code of the .aspx page make sure "AutoPostBack" is set to true, the source code should look like this
<asp:RadioButtonList ID="RadioButtonList1" runat="server"
AutoPostBack="true">
</asp:RadioButtonList>
4. Create a connection string in the Web.Config file
<connectionStrings>5. Create a new folder in your project call "BusinessObjects" then add a .cs file call "Category.cs"
<add name="NorthwindConnectionString" connectionString="Data Source=(local);
Initial Catalog=Northwind;Integrated Security=True"
providerName="System.Data.SqlClient"/>
</connectionStrings>
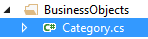
6. In the "Category.cs" file type in the following code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace BusinessObjects
{
public class Category
{
private int _categoryID;
private string _categoryName;
public Category(int Id, string Name)
{
this._categoryID = Id;
this._categoryName = Name;
}
public int CategoryID
{
get { return this._categoryID; }
set { this._categoryID = value; }
}
public string CategoryName
{
get { return this._categoryName; }
set { this._categoryName = value; }
}
}
}
7. In the code behind page(.cs) page of the .aspx page, type in the following lines at the top to use the following libraries
using System.Web.Configuration;8. Create a new method call BindCategoriesRadioButtonListObjects() that returns void
using System.Data.SqlClient;
using System.Data;
using BusinessObjects;
protected void BindCategoriesRadioButtonListObjects()
{
DataTable dtCategories = new DataTable();
string connectString = WebConfigurationManager.ConnectionStrings["NorthwindConnectionString"].ConnectionString;
Listcategories = new List ();
using (SqlConnection conn = new SqlConnection(connectString))
{
SqlCommand cmd = new SqlCommand("SELECT CategoryID,CategoryName FROM Categories", conn);
conn.Open();
SqlDataAdapter adapter = new SqlDataAdapter(cmd);
adapter.Fill(dtCategories);
foreach (DataRow row in dtCategories.Rows)
{
categories.Add(new Category(Convert.ToInt32(row["CategoryID"].ToString()), row["CategoryName"].ToString()));
}
//used to set the RadioButtonList1.DataSource to categories list of objects
RadioButtonList1.DataSource = categories;
RadioButtonList1.DataTextField = "CategoryName";
RadioButtonList1.DataValueField = "CategoryID";
RadioButtonList1.DataBind();
}
}
Comments
Post a Comment